- 좋은 매칭 선별 방법 #1
- 가장 좋은 매칭 결과에서 distance 값이 작은 것 N개를 사용
- cv2.DMatch.distance 값을 기준으로 정렬 후 상위 N개를 선택
import sys
import numpy as np
import cv2
# 영상 불러오기
src1 = cv2.imread('graf1.png', cv2.IMREAD_GRAYSCALE)
src2 = cv2.imread('graf3.png', cv2.IMREAD_GRAYSCALE)
if src1 is None or src2 is None:
print('Image load failed!')
sys.exit()
# 특징점 알고리즘 객체 생성 (KAZE, AKAZE, ORB 등)
#feature = cv2.KAZE_create()
#feature = cv2.AKAZE_create()
feature = cv2.ORB_create()
# 특징점 검출 및 기술자 계산
kp1, desc1 = feature.detectAndCompute(src1, None)
kp2, desc2 = feature.detectAndCompute(src2, None)
# 특징점 매칭
# matcher = cv2.BFMatcher_create()
matcher = cv2.BFMatcher_create(cv2.NORM_HAMMING)
matches = matcher.match(desc1, desc2)
# 좋은 매칭 결과 선별
matches = sorted(matches, key=lambda x: x.distance)
good_matches = matches[:80]
print('# of kp1:', len(kp1))
print('# of kp2:', len(kp2))
print('# of matches:', len(matches))
print('# of good_matches:', len(good_matches))
# 특징점 매칭 결과 영상 생성
dst = cv2.drawMatches(src1, kp1, src2, kp2, good_matches, None)
cv2.imshow('dst', dst)
cv2.waitKey()
cv2.destroyAllWindows()
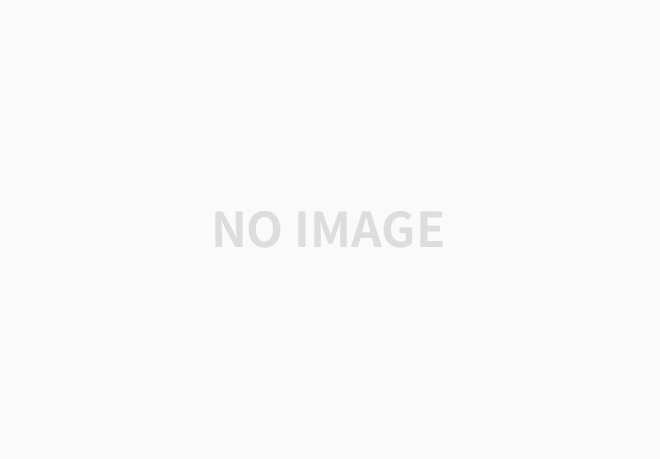
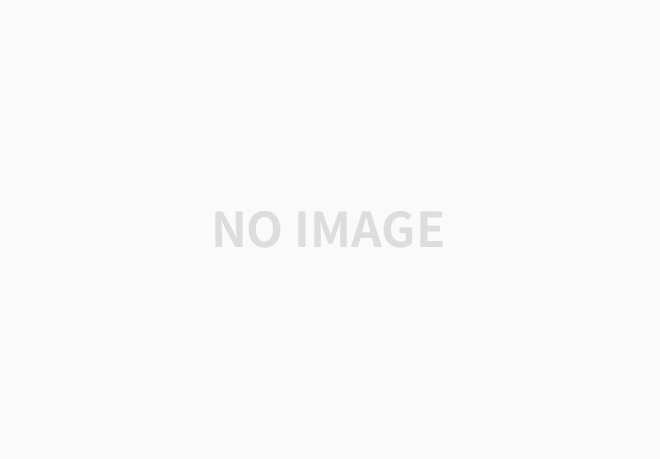
- 좋은 매칭 선별 방법#2
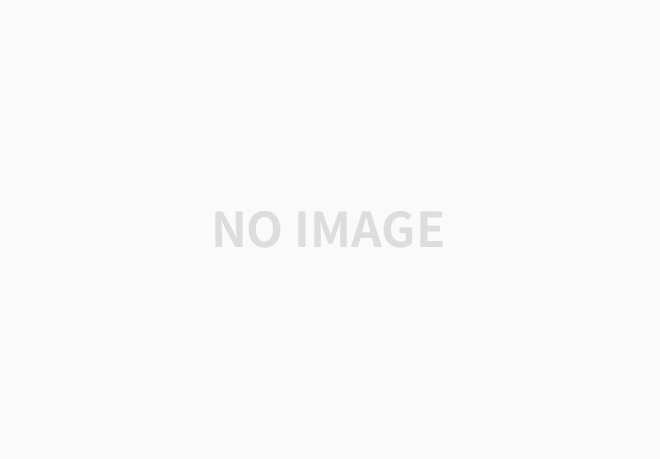
import sys
import numpy as np
import cv2
# 영상 불러오기
src1 = cv2.imread('graf1.png', cv2.IMREAD_GRAYSCALE)
src2 = cv2.imread('graf3.png', cv2.IMREAD_GRAYSCALE)
if src1 is None or src2 is None:
print('Image load failed!')
sys.exit()
# 특징점 알고리즘 객체 생성 (KAZE, AKAZE, ORB 등)
feature = cv2.KAZE_create()
#feature = cv2.AKAZE_create()
#feature = cv2.ORB_create()
# 특징점 검출 및 기술자 계산
kp1, desc1 = feature.detectAndCompute(src1, None)
kp2, desc2 = feature.detectAndCompute(src2, None)
# 특징점 매칭
matcher = cv2.BFMatcher_create()
#matcher = cv2.BFMatcher_create(cv2.NORM_HAMMING)
matches = matcher.knnMatch(desc1, desc2, 2)
# 좋은 매칭 결과 선별
good_matches = []
for m in matches:
if m[0].distance / m[1].distance < 0.7:
good_matches.append(m[0])
print('# of kp1:', len(kp1))
print('# of kp2:', len(kp2))
print('# of matches:', len(matches))
print('# of good_matches:', len(good_matches))
# 특징점 매칭 결과 영상 생성
dst = cv2.drawMatches(src1, kp1, src2, kp2, good_matches, None)
cv2.imshow('dst', dst)
cv2.waitKey()
cv2.destroyAllWindows()
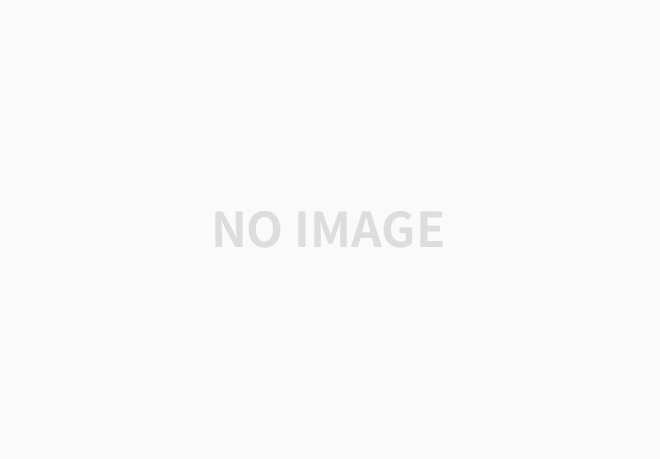
'Computer Vision > opencv(python)' 카테고리의 다른 글
[61] 7) 이미지 스티칭 (0) | 2021.03.25 |
---|---|
[60] 6) 호모그래피와 영상 매칭 (0) | 2021.03.25 |
[58] 4) 특징점 매칭 (0) | 2021.03.25 |
[57] 3) 특징점 기술자 (0) | 2021.03.25 |
[56] 2) 특징점 검출 (0) | 2021.03.25 |